100 Days of Python
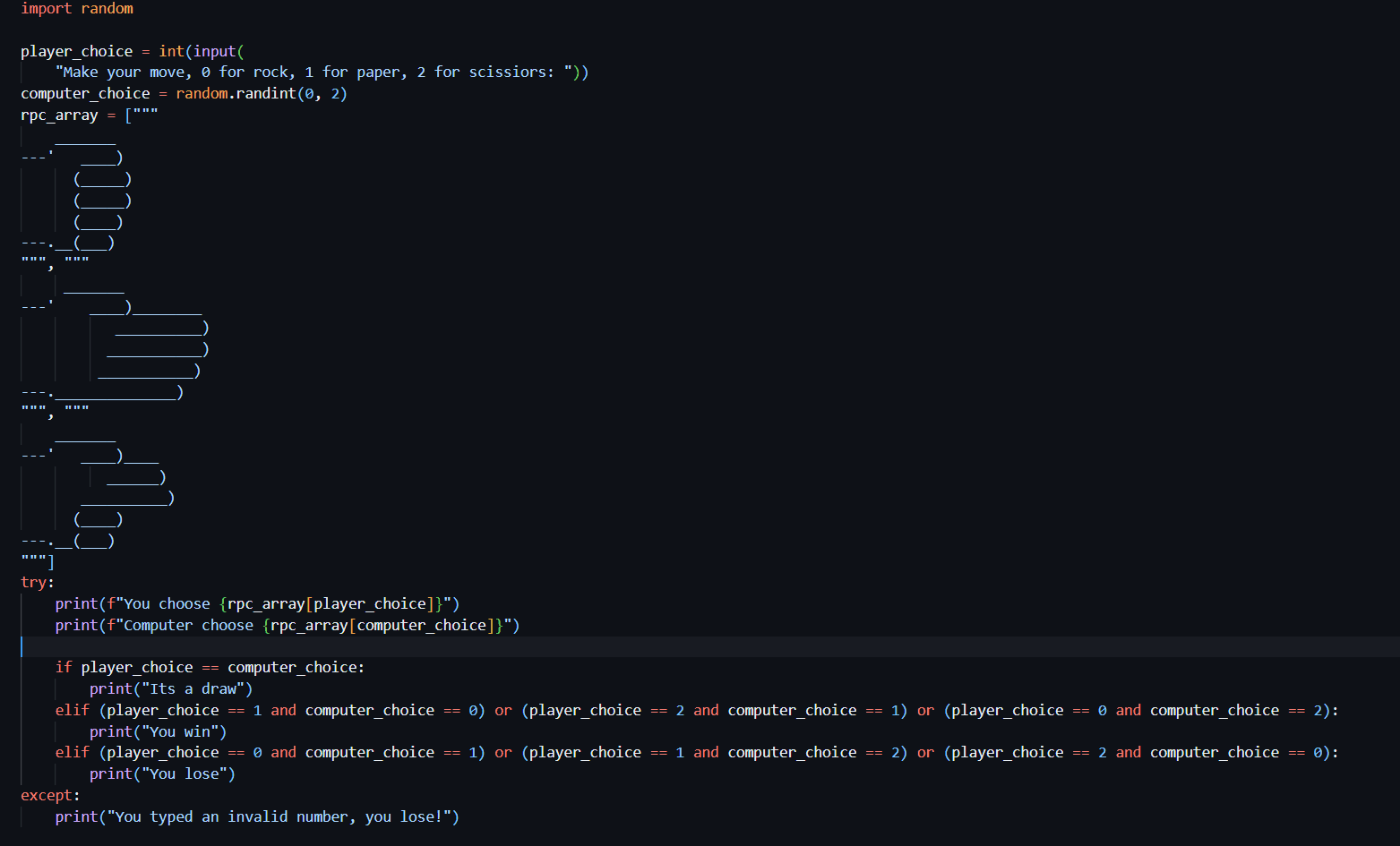
About 50 projects from ANgela Yu's 100 Days of Python course. Projects include games, automation, web scraping, and more.
Mastering Python Through Projects: A Journey Through 100 Days of Code
Learning to code is a journey best undertaken one step at a time, with consistent practice and real-world applications. The "100 Days of Python" challenge embodies this philosophy perfectly, guiding learners from basic syntax to complex web applications through daily coding projects. Let's explore an impressive repository documenting one developer's journey through this comprehensive Python bootcamp.
The Learning Path: From Print Statements to Web Apps
Looking through this repository reveals a carefully structured progression of skills:
Foundation Phase (Days 1-10)
The repository begins with simple yet engaging projects that build core Python fundamentals:
- Day 1: Band Name Generator - A simple program combining user inputs to create band names, introducing variables and string concatenation
- Day 2: Tip Calculator - Processing numerical inputs and performing calculations
- Day 3: Choose Your Adventure Game - Using conditional statements to create branching storylines
- Day 4: Rock Paper Scissors - Implementing game logic with conditionals and random number generation
- Day 5: Password Generator - Working with loops and randomization
- Day 7: Hangman - A classic word game using lists and string manipulation
- Day 8: Caesar Cipher - Encrypting messages by shifting letters, demonstrating more advanced string manipulation
Intermediate Concepts (Days 11-20)
Projects grow more sophisticated, introducing functions, dictionaries, and object-oriented programming:
- Day 10: Calculator - Creating a functional calculator with multiple operations using dictionaries to map operations to functions
- Day 11: Blackjack - Implementing a complete text-based card game
- Day 14: Higher Lower Game - Comparing social media followers in a guessing game
- Day 16: Coffee Machine OOP - Reimagining procedural code using classes and objects
- Day 17: Quiz Game - Building a full quiz application with OOP principles
- Day 18-19: Turtle Graphics Projects - Creating visual art and games with Python's turtle module
Advanced Python (Days 20-30)
Here we see projects employing external libraries and more complex programming concepts:
- Day 20-21: Snake Game - A complete game built with OOP design patterns
- Day 25: Pandas Data Analysis - Working with CSV data and the Pandas library
- Day 26: List & Dictionary Comprehensions - Advanced data manipulation techniques
- Day 27-28: Tkinter GUI Applications - Building desktop applications with Python
Professional Applications (Days 30+)
The final phase moves into real-world applications that could serve as portfolio pieces:
- Day 33-34: API Projects - Working with APIs to fetch data from external services
- Day 37: Habit Tracker - Building applications that persist data
- Day 54-57: Web Development with Flask - Creating web applications with routing and templates
Deep Dive: Notable Projects
Rock Paper Scissors Game (Day 4)
This project stands out for its clever use of ASCII art to represent hand gestures:
The game includes error handling to prevent crashes and clear win/loss logic:
Snake Game (Day 20-21)
The repository implements the classic Snake game with proper OOP architecture, separating concerns into distinct classes:
- Snake class for managing the snake's body and movement
- Food class for generating random food items
- Scoreboard class for tracking and displaying the score
This modular approach demonstrates software engineering best practices applied to game development.
Flask Web Applications (Days 54-57)
By day 57, we see a complete web application using Flask and Jinja templating:
This endpoint connects to external APIs to guess a user's gender and age based on their name, then uses Jinja templates to render the results—showcasing how far the developer has come from simple console applications.
The Project-Based Learning Advantage
What makes this repository remarkable is how it exemplifies effective learning through projects:
- Progressive Complexity: Each project builds upon previously learned concepts while introducing new ones
- Practical Application: Abstract concepts are immediately applied to real problems
- Variety of Domains: Games, tools, data analysis, web applications—providing exposure to different use cases
- Modular Development: Breaking down complex applications into manageable components
- Technology Integration: Working with external libraries, APIs, and frameworks
Learning Patterns and Concepts
Throughout the repository, we see documentation of key programming concepts:
- Functions and Decorators: From basic functions to advanced Python decorators
- Object-Oriented Programming: Classes, inheritance, and encapsulation
- Data Structures: Lists, dictionaries, and more advanced data manipulation
- File I/O: Reading from and writing to files
- API Integration: Making HTTP requests and processing JSON responses
- Web Development: Server-side rendering with Flask and Jinja
Tracking Progress and Setting Goals
The repository includes a Targets.md file showing the developer's commitment to consistent progress:
This approach of setting clear, achievable goals likely contributed to maintaining momentum throughout the challenge.
Conclusion
This "100 Days of Python" repository demonstrates the power of consistent, project-based learning. Starting with simple scripts and progressively tackling more complex challenges, the developer has built an impressive portfolio of Python applications spanning various domains.
The journey from printing "Hello World" to building web applications that integrate with external APIs illustrates how far one can progress with disciplined daily practice. Each project serves not just as a learning exercise but as tangible proof of growing skills.
For anyone considering learning Python—or any programming language—this repository provides an inspiring blueprint: start small, be consistent, build real projects, and gradually increase complexity as your skills grow. The results, as demonstrated here, can be remarkable.